Brightcove Interactivity provides programmatic access to the reporting data shown in the Studio Reports user interface. This document provides details on how to access the API containing that data from a server-side process like Python or cURL.
The basics
Brightcove Interactivity provides programmatic access to reporting data through two sets of API endpoints
Engagement Reports Base URL
https://interactivity-reporting.api.brightcove.com/api/reports/v2/
Analytics Reports Base URL
https://interactivity.api.brightcove.com
Authenticating requests
The reporting API uses Brightcove's OAuth system for authentication, using the client credential flow. This means that you obtain client credentials (a client_id
and client_secret
) and use those to obtain an access token which is submitted in the Authorization header like this:
Authorization: Bearer access_token_here
To generate the Access Token, see the information on Getting Access Tokens.
The easiest way to get client credentials is through Studio - see Managing API Credentials. The permissions you need for Interactivity are:
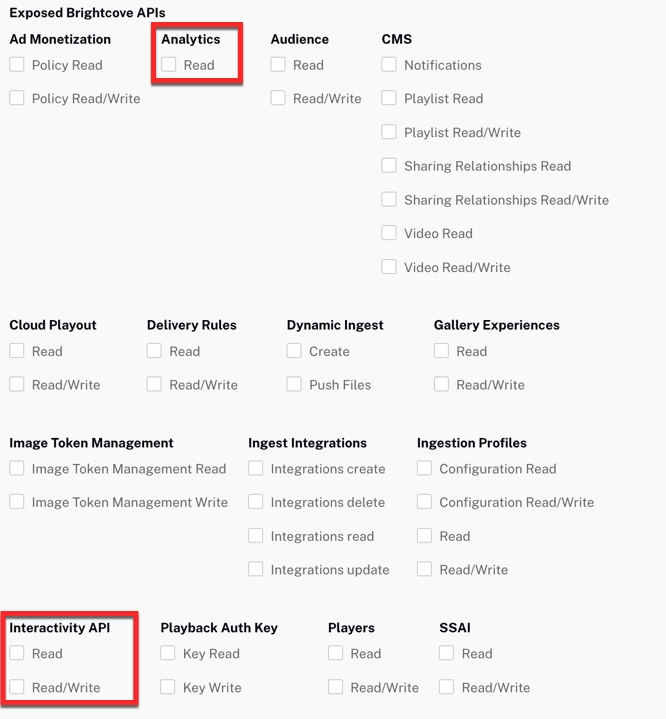
You can find your own reporting API key on the integrations tab of the admin section of the Brightcove Interactivity Portal. Treat your reporting API key with the sensitivity of a password as anyone with it can use it to retrieve reporting data from your account.
Base URL for reporting requests:
Example URL structure
https://[base-url]/[report-name]?send_status=true&[parameters]
Example request
interactivity-reporting.api.brightcove.com/api/reports/v2/project_engagement_summary_raw?send_status=true&account_id=6415650835001
API request construction
The API is invoked via GET operation to the Base API URL. The request must contain a valid Authorization
header. Specific reports are requested by appending the name of the report and valid parameters to the Base API URL.
Results are not synchronously returned. The reporting API operation is asynchronous in that results may not be available when your request returns. In this case your request will be queued and you will need to poll, or try later, for results.
Requests must always include send_status=true
which forces the API to return HTTP 202 status while the reporting request is processing and then HTTP 200 with results when it is complete.
To determine if you need to "poll" for results, examine the HTTP status code returned by the API. If it is "202 Accepted" your report is still being generated and you’ll need to repeat the request a little bit later to retrieve results. The results are returned with an HTTP 200 status code when they are complete. Once complete, reporting results are cached for one day and are returned immediately with each request.
Engagement Reports endpoints
Retrieve the following reports via API through the base URL: https://interactivity-reporting.api.brightcove.com/api/reports/v2/
Name | Description | End Point |
---|---|---|
Project Summary | One row per Project | project_engagement_summary_raw |
User Summary | One row per User | user_engagement_summary_raw |
Experience Summary | One row per Experience | experience_engagement_summary_raw |
Poll Widget Summary | One row per Poll Widget instance, per poll_widget_summary response value with count of response values. Project IDs will contain a list of all projects where the poll appeared and was submitted. This is included to support templates where the same poll widget will appear with multiple projects. Note: The Project IDs list is not de-duped. |
poll_widget_summary |
Sign In Widget Summary |
One row per Sign In Widget instance with counts of various Sign In stats. Project IDs will contain a list of all projects where the Sign In appeared and was submitted. This is included to support templates where the same Sign In widget will appear with multiple projects. Note: The Project IDs list is not de-duped. |
signin_widget_summary |
User Activity Stream |
One row per Action, with User ID if known *Includes "views" of bookmarks, user interactions with player controls, annotations and widgets. **Excludes "progress" events. |
user_stream_raw |
Project Activity Stream | One row per Action *Includes "views" of bookmarks, user interactions with player controls, annotations and widgets. **Excludes "progress" events. |
project_stream_raw |
Experience Stream | One row per Action *Includes "views" of bookmarks, user interactions with player controls, annotations and widgets. **Excludes "progress" events. |
experience_stream_raw |
Poll Widget Detail | One row per poll submit event. | poll_widget_detail |
Sign In Widget Detail | One row per Sign In submit event. Includes events where the user was silently signed-in (auto) because of a previous sign-in (manual). | signin_widget_detail |
Chapter Summary Report | One row per chapter annotation. | chapter_summary |
User Sentiment Widget | One row per Project | widget_user_sentiment_summary |
Engagement Report parameters
All Engage Report end_points accept the following parameters:
start
Start date for report results. Default value is 7 days before "end" date. Format for dates is YYYY-MM-DD. All times are UTC.
end
End date for report results. Default value is end of current day. For example if today is 2021-09-23, the default end date is 2021-09-23 23:59:59.999.
format
Output format for report results. Supported values are: csv and json.
send_status
For easier interaction with the API we suggest that you always include send_status=true
. This forces the API to return HTTP 202 status while the reporting request is processing and then HTTP 200 with results when it is complete.
Analytics Reports endpoints
Retrieve the following reports via API through the base URL: https://interactivity.api.brightcove.com
Report Name | Description | Endpoint Path |
---|---|---|
Gradebook Summary Report | Retrieves counts of correctly answered questions grouped by user ID | /v1/accounts/{account_id}/reports/gradebook/summary |
Gradebook User Answer Report | Retrieves user submitted answers | /v1/accounts/{account_id}/reports/gradebook/users/answers |
Quiz Actions Report | Retrieves counts of answered questions grouped by project | /v1/accounts/{account_id}/reports/quiz/actions |
Quiz Project Summary Report | Retrieves counts of unique answers submitted, grouped by question and answer | /v1/accounts/{account_id}/reports/quiz/project/summary |
Analytics Report parameters
For a full list of parameters and specific details for each endpoint, refer to Interactivity API Reference.
Below are common parameters for some endpoints:
account_id
The unique identifier for the account requesting the report.
project_id
Filter results by project ID.
start_time
andend_time
Define the time range for the report.
user_id
Optional fields for retrieving user-specific quiz data.
Important details: limits
- Maximum row count - The API limits the returned results to 500,000 rows of output. Output beyond this limit is omitted from the results.
- Maximum time range - The API limits the time range of data retrieved to six months.
- Rate limits - By default, the reporting API limits you to running 4 reports per minute; 20 per hour; and 100 per day. These limits apply to the number of unique reports you can execute, not the number of times you hit the reporting endpoint to retrieve results or check status on an already queued report.
Python code example
The example below illustrates how to invoke a report from Python using the popular Requests library.
import requests
import sys
import time
TIMEOUT = 120 # consider a report "timed out" if running > 2
minutes = True
processing = True
BASE_DOMAIN= "https://interactivity-reporting.api.brightcove.com/api/reports/v2/"
url = BASE_DOMAIN+"api/reports/v2/project_engagement_summary_raw"
print(url)
# Reporting endpoint url
querystring = {
"start": "2023-07-01",
"end": "2024-03-31",
"format": "csv", # csv or JSON
"send_status":"true"
}
headers = {
'Authorization': "Bearer {Brightove Access Token}",
'cache-control': "no-cache",
}
start_time = time.time()
while processing:
if time.time() - start_time > TIMEOUT:
print('Report timed-out')
sys.exit(-1)
response = requests.request("GET", url, headers=headers, params=querystring)
print('Result: {}'.format(response.status_code))
if response.status_code == 200:
processing = False
print(response.text)
elif response.status_code == 202:
print('sleep/recheck')
time.sleep (10)
else:
print('Something went wrong')
sys.exit(-2)