Overview
Brightcove's implementation consists of two parts:
-
The OAuth API - provides access to all available OAuth functionality
-
The OAuth Credentials UI - accessible through the Account Settings interface in Studio, the UI provides an easy way to register apps that will use Brightcove APIs and generate a client ID and client secret for them. See Managing API Authentication Credentials for instructions on using the OAuth UI.
Also see the API Reference.
Glossary of terms
- OAuth
-
An open standard for authorization. OAuth provides client applications a 'secure delegated access' to server resources on behalf of a resource owner. OAuth essentially allows access tokens to be issued to third-party clients by an authorization server, with the approval of the resource owner. The client then uses the access token to access the protected resources hosted by the resource server
- scope
-
A data object describing a set of resources (accessible via an API) and some operations on those resources (such as "read" and "write"). The scope of an access token limits what you can do by presenting that token.
- client
-
An app used by an end user to access a resource via a Brightcove API.
- client id
-
A unique identifier for a client generated by the OAuth service.
- client secret
-
A string of bits that, used with a client id, serves as a password to authenticate a client.
- access token
-
A string of bits that provides temporary access to an API. Access tokens are returned by the OAuth service for a client on request.
- flow
-
Sequence of operations that results in successfully accessing an OAuth-protected resource.
Base URL
The base URL for the OAuth API is:
https://oauth.brightcove.com/v4
Client credential flow
In the client credential flow, your app will make a request for an access token, passing your client id and client secret to the OAuth service with the request. Currently, this is the only flow supported for Brightcove customers.
Exactly how the client credential flow will work will depend on the scenario.
Organizational app
In this scenario, you have an app that needs to interact with one or more Brightcove APIs, only for the account or accounts that belong to your organization. The app is not tied to any specific user. In this case the workflow looks like this:
To implement this scenario, you would do the following:
-
Using the OAuth UI or the OAuth Service, get a client id and secret for your app - the UI allows you to get a client id and secret for single account or multiple ones. This is a one-time operation.
-
Add logic to your server-side app to make requests to the OAuth API for access tokens. Implementation will depend on the language of your app (and you are encouraged to use an existing OAuth2 library for your language if possible), but the call you will make will be a POST request to:
https://oauth.brightcove.com/v4/access_token
The
client_id
andclient_secret
are passed as theusername:password
in a basic Authorization header:Authorization: Basic {client_id}:{client_secret}
The entire
{client_id}:{client_secret}
string must be Base64 encoded (in Node.js, for example, you can use theBuffer.toString("base64")
method). CURL does the BASE64 encoding automatically, so you can just pass the credentials asuser {client_id}:{client_secret}
. You also need to include aContent-Type: application/x-www-form-urlencoded
header.The request body will contain the key/value pair
grant_type=client_credentials
. Note that since theContent-type
isx-www-form-urlencoded
, you can also just append this to the request URL as a parameter:https://oauth.brightcove.com/v4/access_token?grant_type=client_credentials
Below is a very basic Node.js app that will get an
access_token
given a validclient_id
andclient_secret
./* * Simple node app to get an access_token for a Brightcove API * You will need to substitute valid client_id and client_secret values * for {your_client_id} and {your_client_secret} */ var request = require('request'); var client_id = "{your_client_id}"; var client_secret = "{your_client_secret}"; var auth_string = new Buffer(client_id + ":" + client_secret).toString('base64'); console.log(auth_string); request({ method: 'POST', url: 'https://oauth.brightcove.com/v4/access_token', headers: { 'Authorization': 'Basic ' + auth_string, 'Content-Type': 'application/x-www-form-urlencoded' }, body: 'grant_type=client_credentials' }, function (error, response, body) { console.log('Status: ', response.statusCode); console.log('Headers: ', JSON.stringify(response.headers)); console.log('Response: ', body); console.log('Error: ', error); });
-
The response body will look like the following:
{ "access_token": "ACikM-7Mu04V5s7YBlKgTiPu4ZO3AsTBlWt-73l5kXRN4IeRuIVlJHZkq_lFQdZBYfzT9B_nHNgcmNFdalxSiNdqOBaaV7wQCCnRCua_efHNCg9d_yLbezcjxr3AGcBKy9a8_t-uTMTA46T24LKMOBGBNJFkyATSZI4JuxU71VIyGF9hDisbKHmKC5G5HdQ0nJgyCD1w1zkKrB1CpFb5iiBuA_XOzehF-Xf5DBYnSkDhzzByuFwTv9dU9d7W6V2OuiKiTzCzY3st01qJTk6-an6GcAOD4N5pdN8prvvMDQhz_HunJIamvVGqBz7o3Ltw8CFFJMXKQdeOF8LX31BDnOvMBEz-xvuWErurvrA0r6x5eZH8SuZqeri4ryZAsaitHiJjz9gp533o", "token_type": "Bearer", "expires_in": 300 }
You will need to capture the
access_token
. Unless your calls will be intermittent, in which case you will request a newaccess_token
for every API call, you will also want to capture theexpires_in
value so that you can use it for later requests to check whether your token is still valid - if not, you will need to request a new one. Theexpires_in
value is in seconds. -
Once you have the
access_token
, you can call the Brightcove API, including the token in theAuthorization
header in the form:Authorization: Bearer {access_token}
See Getting Access Tokens for more details and code samples.
General Authorization
This scenario applies mainly to Brightcove partners who will be creating apps that may be used by Brightcove users in various organizations. The workflow for this scenario looks like the following:
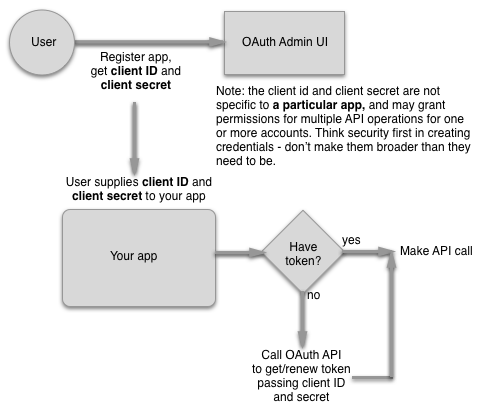
The only difference in implementing this scenario rather than the first one is that the user must get a client id and secret for your app from the OAuth UI and provide these to you via a form. You will then pass these to your app to submit with the request for an access_token
. Aside from that, everything will be the same.
Get Client Credentials
The easiest way to get client credentials (the client_id
and client_secret
) is to use the OAuth UI. If you prefer to get them directly from the OAuth Service however, you can do so by making a POST request to https://oauth.brightcove.com/v4/client_credentials
, passing the following headers:
Content-Type: application/json
Authorization: BC_TOKEN your BC_TOKEN
You also need to send a JSON object as the payload:
{
"type": "credential",
"maximum_scope": [
{
"identity": {
"type": "video-cloud-account",
"type": "perform-account",
"account-id": account_id1
},
"operations": [
"video-cloud/player/all"
]
},
{
"identity": {
"type": "video-cloud-account",
"type": "perform-account",
"account-id": account_id2
},
"operations": [
"video-cloud/player/all"
]
}
],
"name": "AnalyticsClient",
"description": "My analytics app"
}
Operations
The only thing that will vary here is the operations
value, which will depend on which API you want access to, and whether you want access to read, write, or both operations. See API Operations for Client Credentials Requests for a list of all operations currently supported.
For detailed guides to getting client credentials using curl or Postman, see:
Working with OAuth
To build logic to handle getting access tokens for your API requests, there are two general ways to proceed.
If you are building a single server-side app, it makes sense to build the logic into the app.
If instead you will be building multiple apps that will need make calls to Brightcove APIs, or if you creating a client-side web app, it makes more sense to consolidate the code for getting access tokens into a single proxy.
See the Quick Start for detailed instructions on creating a simple proxy.
Client samples and libraries
We have created sample client implementations in several languages to give you models for implementations.
There are also OAuth2 libraries available for a number of languages, and we generally encourage you to use these libraries where possible, rather than building interaction with the OAuth API. Below is a partial list of available libraries. For a more extensive list, see https://oauth.net/2/
- Python
- PHP
- Cocoa
- Cocoa
- iOS
- iPhone and iPad
- iOS and Mac MacOS
- iOS and Mac MacOS
- Java
- Ruby
- .NET
- Qt/C++
- O2
Known Issues
- Currently, Brightcove has a limitation of 30 accounts per client credential. If you exceed that limit for one client credential, you will not see the accounts to which this credential belongs, it is no longer possible to see any credentials in any accounts. This affects both the user interface and the underlying. For more information, see Get Client Credential. However, in this case, it only affects the ability to see credential details. The credentials still exist and function, creating tokens as usual. A way to see the credentials visible is that there is no longer a single credential assigned to more than 30 accounts.