Introduction
Cue points are specified places on the video timeline. When the video is played back, an event is automatically broadcast every time a cue point is reached - using the Player API, you can listen for these events and take whatever action you wish. In addition, you can read all the cue point information from the video metadata stored in the player mediainfo
object, which allows you to mark the cue points on the video timeline in some way if you want to.
There are two kinds of cue points:
- Ad cue points: these are used to mark places where an ad request should be made (if the video and player are enabled for advertising).
- Code cue points: these are used to take some action using the Player API - some typical uses are displaying some kind of call-to-action (CTA) or displaying some image or text relating to video content, perhaps as a video overlay or in the page around the player.
Sample app
Find all the code associated with this sample in this GitHub repository.See the Pen CMS API Sample: Add Cue Points by Brightcove Learning Services ( @rcrooks1969) on CodePen.
Implementation overview
You can add cue points in the Studio Media module. You can also add them using the CMS API, which is the method we will use in this sample.
HTTP method
Cue points can be added either when you create the video or later with an update request. In this sample, we will add them to an existing video by making a video update request, which means you will use the PATCH
method.
Endpoint
The endpoint for the update video request is:
https://cms.api.brightcove.com/v1/accounts/account_id
/videos/video_id
Request body
The request body is a JSON object that can contain many items of video metadata. Here we will just look at the cue_points
field, which is set to an array of cue point objects, as in the following sample:
{
"cue_points": [
{
"name": "Ad Cue Point",
"type": "AD",
"time": 2.0,
"metadata": null,
"force_stop": false
},
{
"name": "Code Cue Point",
"type": "CODE",
"time": 4.0,
"metadata": "The metadata might be text or the url for an image to display",
"force_stop": true
}
]
}
Cue point object fields
Below is a description of the fields for cue point objects.
Field | Type | Required | Description |
---|---|---|---|
name |
string | no | An arbitrary name - this may be useful to identify a particular cue point in your Player API code |
type |
string | yes | Must be AD or CODE |
time |
number | yes | Time for the cue point in seconds from the beginning of the video [1] |
metadata |
string | no | Any string up to 128 single-byte characters - this could be information useful to your cue point event handler, such as the text for an overlay or the location of an image to display |
force_stop |
boolean | no | Whether the video should be paused when the cue point is reached ( force_stop is ignored by the Brightcove Player and there are no plans currently to support it, but you can pause the video manually in your cue point event handler) |
Notes
- [1] Technically, the cue point is active until the next cue point is reached - therefore, in the cue points array that you can retrieve from the Brightcove Player, you will see a
startTime
andendTime
as well at atime
for the cue point.
Getting Authentication Credentials
To use the CMS API you will need proper credentials.
The easiest way to get credentials in most cases is through the Studio Admin API Authentication section (requires admin permissions on your account). See Managing API Authentication Credentials for details. In most cases, you probably just want to get permissions for all CMS API operation:
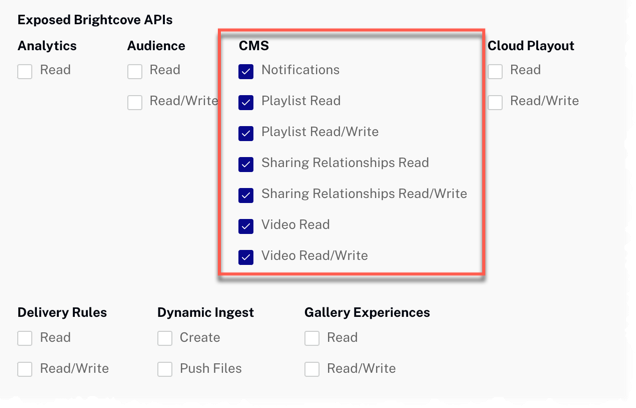
If the permissions you need are not available in Studio, or if you prefer to get them directly from the OAuth API, use your choice of the Get Client Credentials documents listed below. Whichever option you choose, you will need to ask for the correct operation permissions. The following can be used with cURL or Postman to get all permissions for the CMS API:
"operations": [
"video-cloud/video/all",
"video-cloud/playlist/all",
"video-cloud/sharing-relationships/all",
"video-cloud/notifications/all"
]
Using the CodePen
- Toggle the actual display of the app by clicking the Result button.
- Click the HTML/CSS/JS buttons to display ONE of the code types.
- Click Edit on CodePen in the upper right corner to fork this CodePen into your own account.
- Find all the code associated with this sample in this GitHub repository.
Proxy code
In order to build your own version the sample app on this page, you must create and host your own proxy. (The proxies used by Brightcove Learning Services only accept requests from Brightcove domains.) A sample proxy, very similar to the one we use, but without the checks that block requests from non-Brightcove domains, can be found in this GitHub repository. You will also find basic instructions for using it there, and a more elaborate guide to building apps around the proxy in Using the REST APIs.